What would you do without an Internet connection? If you are like me, you will feel stranded pretty soon. This is true for many people, but also for many applications. In fact, most software needs to speak with the Internet to be useful and do their job. In this JavaScript tutorial, we will see how to do just that: handling HTTP (and HTTPS) calls in JavaScript.
As you can guess, in this article we use JavaScript to do something. Thus, you really need to grasp the basics of JavaScript already. In case you don’t, don’t panic – you just need to read this tutorial first.
Understand HTTP(S) Calls
If you already know about HTTP, just skip this section. Otherwise, enjoy the reading.
HTTP stands for Hyper-Text Transfer Protocol, Secure in the case of HTTPS. Other than the encryption layer (which is no small thing), HTTP and HTTPS are the very same. They are a protocol that allows you to transfer some data between two remote PCs. Specifically, one PC – the client – asks the other – the server “Hey, can I get this piece of data?”, and the server gives it back.
After that data exchange, communication finishes. If you need another piece of data or another file, you need to re-open another connection. That is totally fine, our PC can do that in the background to fetch all the files and pieces of data that are needed, as in the picture below.
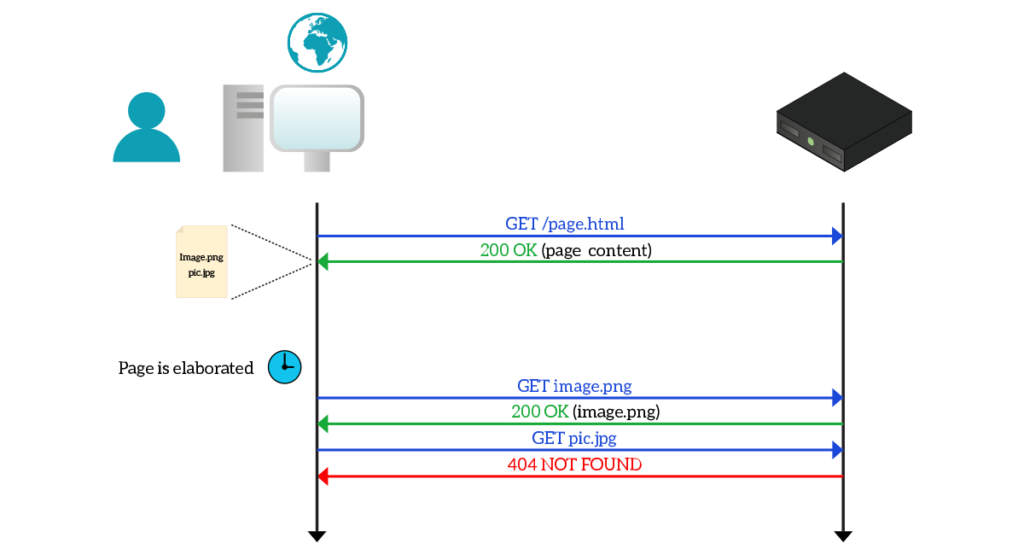
We can use this approach with JavaScript as well. Our application can request some data from a server. For example, we may request some information about the weather, or – more likely – we can build a server on our own that provides the data that we need. That is the backend of an application, while our JavaScript code will be the frontend.
AJAX and JSON
The concept is extremely simple. We just need to get a piece of information from a server. However, that leaves room for other questions. Most notably: how should we format this piece of data?
We know that if we want to create a file that is ready to be displayed to the user, we should go with HTML. But in this case, we want our JavaScript to digest the information and do something with it, so HTML is not the ideal format. Instead, we have two options: XML, and JSON.
XML is the most complex of the two, and the oldest one. Thus, it is not recommended, but just know the whole practice of making HTTP calls with JavaScript started with this format. In fact, the first popular framework to do so was AJAX: Asynchronous Javascript and XML. Besides being cumbersome, it is also not efficient as for every piece of information you carry, you also have to bring with you some metadata that just makes the connection heavier, and slower.
No, the best format to use is JSON. This format is better because it is easier to parse, easier to read, and much lighter. Basically, it is a set of key-value pairs. The key defines what the value is, while the value is the actual value for that property. It is very similar to objects (indeed JSON stands for JavaScript Object Notation). Below, an example.
{
"key": "value",
"first_name": "John",
"last_name": "Doe",
"is_admin": "false",
"roles": [
"editor",
"subscriber",
"contributor",
],
"privacy": {
"required": "true",
"promotional": "false",
"extra": "true",
"notifications": "true",
}
}
HTTP Calls in JavaScript
Making a Request
What we want is simple, a function that receives an URL as a parameter and that returns the obtained file, as a string. Something like httpGet()
.
How do we go implementing this function? We have several options, but we can start with the most vanilla one: AJAX. In fact, even if AJAX was meant to be used with XML, we can use it with any file format.
The first thing we have to do is to define an object that represents the request (req
in our example). Then, we need to provide our URL to it and send the request with no body (we need to receive data, not sending it at the moment). Eventually, we need to return the text that we receive as a response.
function httpGet(uri) { let req = new XMLHttpRequest(); req.open( "GET", uri, false ); // false for synchronous request req.send(null); // no body return req.responseText; }
Note “false for synchronous request”. A synchronous request simply means that we wait for JavaScript to ask the data and get them back before going forward. This may not be ideal in all cases, but it is the simplest option. The other, instead, is asynchronous calls, where we just launch the request and we will handle the response at some point, independent from the execution of the main script.
Parsing the Data
If we receive a JSON file, we can quickly load it into an object. That is possible with the JSON.parse()
function, which takes a string as an argument and returns an object indeed.
In the following example, we fetch the list of ISO countries from a public GitHub repository, and we store them into an object, which in this case it will be an array actually.
let countries = JSON.parse(httpGet('https://raw.githubusercontent.com/lukes/ISO-3166-Countries-with-Regional-Codes/master/all/all.json'));
We can now go on and log them.
for(let i = 0; i < countries.length; i++) { console.log(countries[i]); }
Instead, if we want to convert one of our objects to a JSON string, we can use JSON.stringify()
. That is effectively the opposite of JSON.parse()
, and it is very useful when we want to make a POST request, so when we want to send data to the server. We might want to do that when creating a new account, adding a new invoice in case of an invoice application, and so on.
let countriesString = JSON.stringify(countires);
AXIOS
We know that AJAX is something from the past. AXIOS is from the present. This tiny library is useful to make HTTP requests in a way that is more modern, and easier to read.
The first thing you want to do is to include the AXIOS library among your scripts in the HTML. So, insert the following code in the header.
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
Now, in any other script, we can use the axios
object. With that, we can easily create asynchronous requests. To do that, we can simply use axios.get()
and provide the URL that we want to fetch. This is a Promise, a special JavaScript construct that will return at a later time in the future. On this construct, we can use .then()
, provided with a function. This says “Once you finish there, pass your result to that function”.
axios.get('https://raw.githubusercontent.com/lukes/ISO-3166-Countries-with-Regional-Codes/master/all/all.json').then(function (response) { console.log(response); for(let i = 0; i < response.data.length; i++) { console.log(response.data[i]): } });
Here, the response is already parsed to an object automatically and stored into response.data
.
In addition, we can also use .catch()
to have a special function to handle failures for the request, and another .then()
after that to have some code that is executed regardless of the result, but after the attempt was made.
axios.get('url') .then(function (response) { // Executed on success }).catch(function (error) { // Executed on error }).then(function () { // Executed in any case });
Finally, we can easily post objects in a POST request.
axios.post('url', { firstName: 'John', lastName: 'Doe', }).then(function (response) {});
In case you want to better understand AXIOS, you should check our tutorial on JavaScript Promises.
JavaScript HTTP Requests for Full Stack Developers
Being able to handle HTTP Requests with JavaScript is crucial for any developer, and particularly for Full Stack Developers. In fact, it is not a surprise that is tutorial is part of our entirely free Full Stack Development course.
Since the best way to learn is to try things for yourself, we have an exercise for you. You should try it, it will help you a lot fixing your knowledge. It is also part of our larger pretend bakery store website, which you can find on GitHub.com at alessandromaggio/full-stack-course.
The Assignment
For this assignment, we will need to create a section in our index.html page named "Product of the Day"
. This section must have a paragraph, with an id of #pod
, that should be empty at the start.
Then, in a newly created file named product-of-the-day.js
, we should load it using AXIOS. We should load it from another file that we have to create, pod.json
, which should contain the following.
{
"text": "A fresh loaf of bread with raisins"
}
Our goal is to take that text property and put it inside the paragraph, having a final result that will look pretty much like the one below.
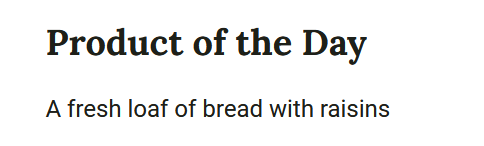
Now, before jumping at the solution below, try as hard as you can figuring out this assignment on your own. Only after that, go check the solution. Is not a complex task after all!
Good luck!
The Solution
So, here’s the solution. Be sure to have tried on your own before checking it. In case you want more info or see the entire project code for this assignment, you should check this commit on GitHub.com.
The first thing we want to do is to load AXIOS, as our assignment requires us to do so. In addition, we also load our product-of-the-day.js
, which we should create in our js
folder. To do all that, we add the following lines inside the header of index.html
.
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script> <script src="js/product-of-the-day.js"></script>
In the same file, we also need to add our paragraph that will host the content that we load. We decided to add it right after the burger buns, so the code that we add is the following.
<h2>Product of the Day</h2> <p id="pod"></p>
Note that we set the ID to pod
as requested in the assignment. Now we are ready to add our JavaScript code inside product-of-the-day.js
.
The code is fairly simple. We fetch the file, and upon success we go fetch the paragraph object by ID and set its inner HTML to the value of text
in the parsed response (which is the response.data
object).
axios.get('pod.json') .then(function (response) { document.getElementById('pod').innerHTML = response.data.text; }).catch(function (error) { console.log(error) });
And that is all! You now have successfully created a script that is able to load content dynamically!
In Conclusion
Fetching content dynamically is a key pillar of modern web development. With that, you can easily create complex pages without the annoying factor of having the page to reload all the time. You can create a web application, not a simple website.
This starts to connect you with the backend, which often is at the other side of those requests. Consider this JavaScript tutorial as a major advancement in your path to become a Full Stack Developer, as HTTP Calls in JavaScript are absolutely needed. But the journey is not complete. We can now work fine-tune what we larned, and then go on with more complex JavaScript, introducing frameworks.
One Response
Comments are closed.